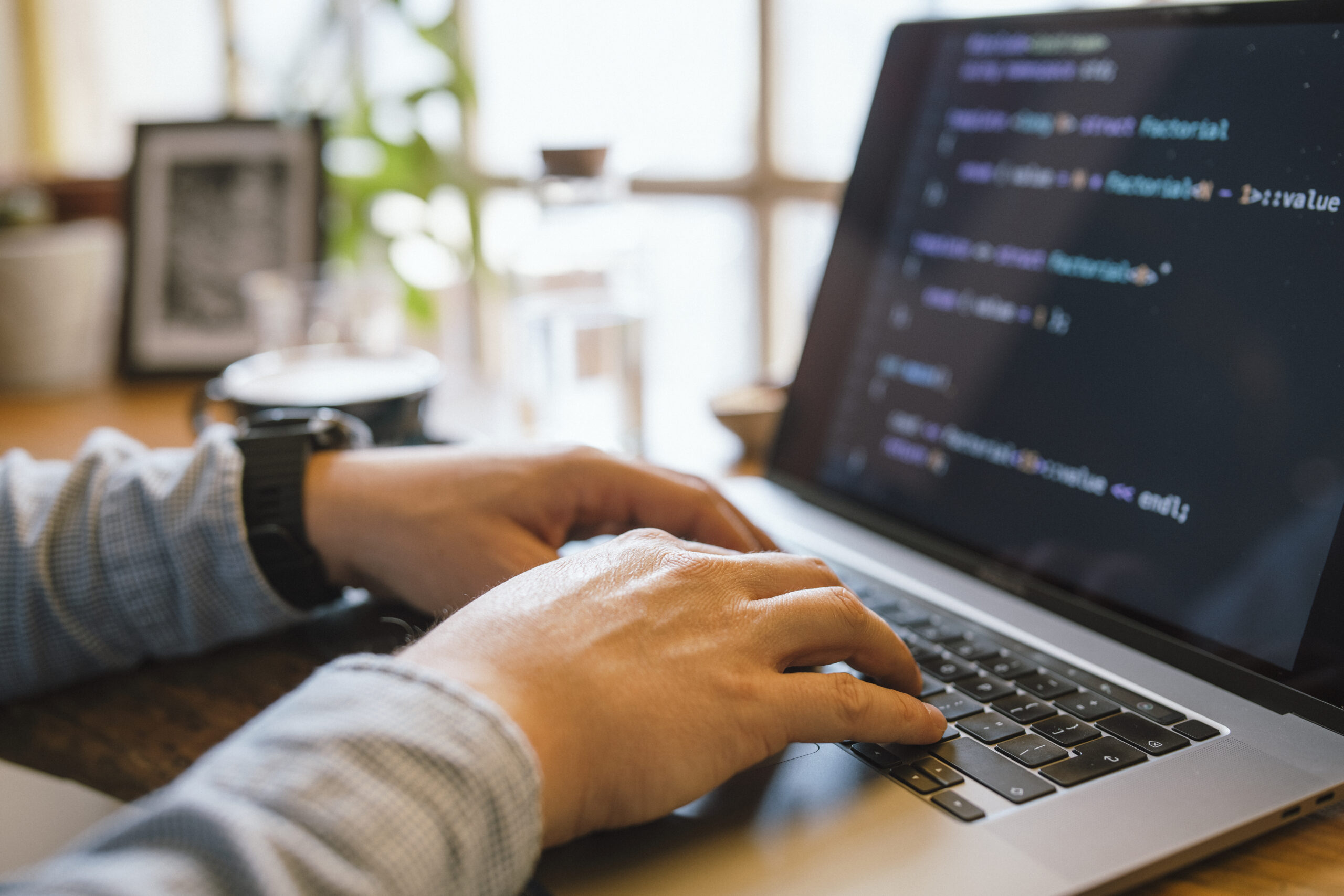
Debugging is Probably the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve difficulties proficiently. No matter if you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can preserve hrs of stress and substantially increase your productiveness. Listed below are a number of methods to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest strategies builders can elevate their debugging expertise is by mastering the tools they use every day. Though producing code is one particular Portion of development, recognizing tips on how to communicate with it successfully during execution is Similarly crucial. Contemporary development environments occur Outfitted with strong debugging capabilities — but lots of builders only scratch the floor of what these resources can do.
Acquire, by way of example, an Integrated Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code over the fly. When utilised properly, they Permit you to observe particularly how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, look at serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can convert irritating UI difficulties into workable duties.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance issues, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into snug with version Manage techniques like Git to be aware of code record, find the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your resources implies heading over and above default options and shortcuts — it’s about producing an personal familiarity with your enhancement ecosystem to ensure that when problems come up, you’re not misplaced at midnight. The better you understand your resources, the more time you are able to invest solving the actual trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to produce a reliable setting or situation where the bug reliably seems. With no reproducibility, fixing a bug becomes a video game of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve gathered adequate information and facts, try and recreate the problem in your local environment. This might necessarily mean inputting the identical data, simulating equivalent person interactions, or mimicking program states. If The difficulty appears intermittently, contemplate crafting automated assessments that replicate the sting instances or condition transitions associated. These exams not simply help expose the trouble but will also stop regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain working devices, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be now midway to correcting it. Which has a reproducible scenario, You should use your debugging resources much more efficiently, examination likely fixes securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it happened, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the concept cautiously As well as in total. Numerous builders, particularly when under time force, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs could lie the legitimate root lead to. Don’t just copy and paste mistake messages into engines like google — study and fully grasp them very first.
Crack the error down into pieces. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can information your investigation and point you toward the dependable code.
It’s also useful to be aware of the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can significantly accelerate your debugging system.
Some mistakes are obscure or generic, and in People conditions, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger challenges and provide hints about possible bugs.
Eventually, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is Among the most highly effective applications in a developer’s debugging toolkit. When utilised correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move from the code line by line.
A fantastic logging tactic starts off with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Data for basic occasions (like successful get started-ups), Alert for likely concerns that don’t break the applying, Mistake for real issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on vital functions, state variations, input/output values, and critical choice details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot concerns, attain deeper visibility into your programs, and Enhance the Over-all maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully recognize and deal with bugs, builders must strategy the method just like a detective resolving a secret. This mindset assists break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Just like a detective surveys a crime scene, collect just as much relevant information as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request on your own: What may very well be causing this habits? Have any alterations just lately been manufactured on the codebase? Has this situation occurred prior to under similar instances? The target is usually to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Try and recreate the challenge inside a managed ecosystem. In case you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the final results lead you nearer to the reality.
Shell out close awareness to tiny details. Bugs normally conceal in the minimum expected destinations—like a lacking semicolon, an off-by-1 error, or simply a race affliction. Be extensive and affected person, resisting the urge to patch The difficulty with no fully knowledge it. Temporary fixes may well hide the true trouble, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and assist Other folks have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complex techniques.
Produce Checks
Writing exams is one of the best solutions to improve your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety Internet that provides you self confidence when building variations towards your codebase. A very well-analyzed software is simpler to debug as it means that you can pinpoint accurately where by and when a problem takes place.
Get started with device checks, which center on unique capabilities or modules. These smaller, isolated checks can promptly expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to look, significantly lessening some time expended debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Upcoming, combine integration exams and end-to-close assessments into your workflow. These aid make sure that many portions of your application do the job jointly easily. They’re particularly practical for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to definitely Believe critically regarding your code. To test a aspect appropriately, you need to be aware of its inputs, expected outputs, and edge scenarios. This level of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing check that reproduces the bug is often a powerful initial step. When the test fails persistently, you could give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s quick to be immersed in the issue—looking at your display for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is solely stepping absent. Having breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're as well close to the code for too lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you simply wrote just hours before. During this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different task for ten–15 minutes can refresh your target. Numerous builders report getting the basis of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It gives your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you face is a lot more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a little something valuable should you make time to replicate and assess what went wrong.
Begin by asking oneself a number of critical issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Create more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively stay away website from.
In group environments, sharing what you've acquired from the bug using your peers is usually In particular strong. No matter whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts staff effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you deal with adds a whole new layer towards your skill established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and tolerance — but the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.